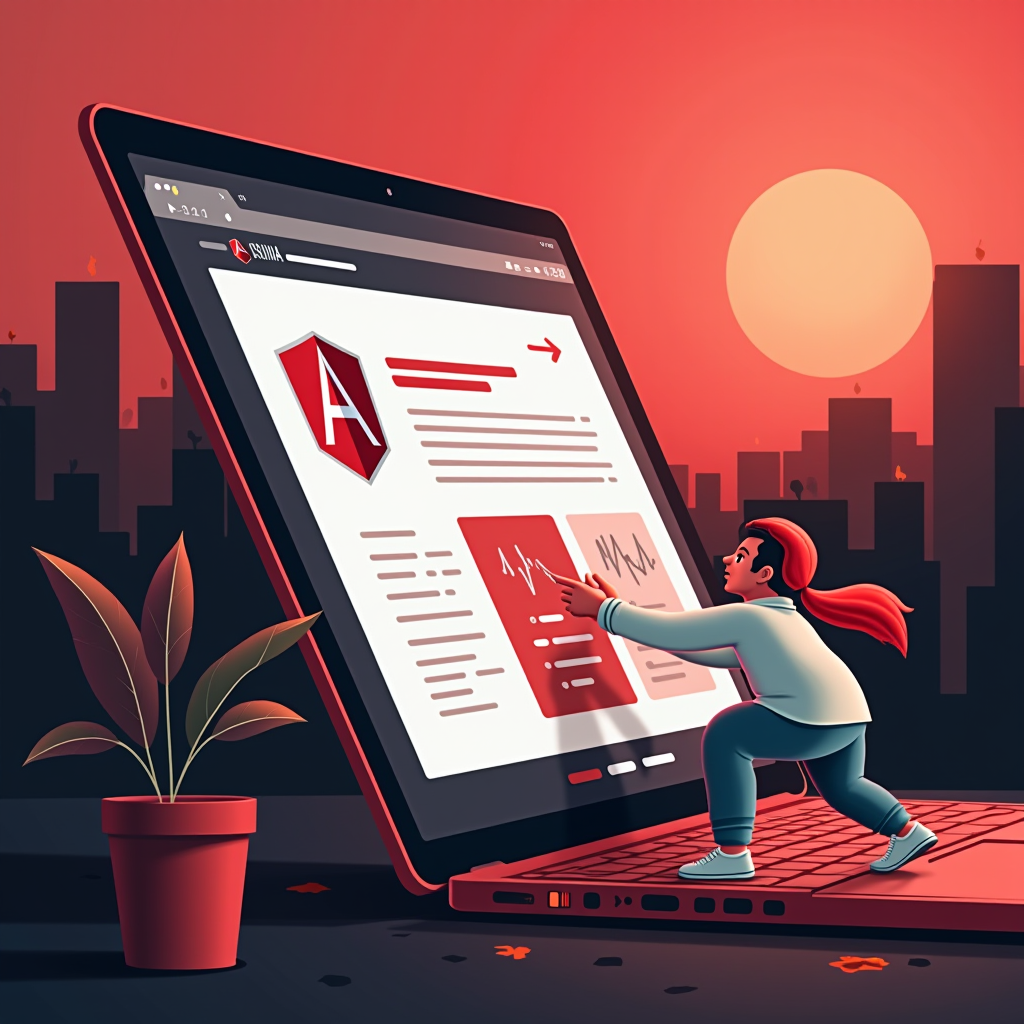
Optimizing Angular 18 Applications with Lazy Loading
Introduction: Lazy Loading in Angular
Angular applications can grow large as more features are added, leading to longer load times. Lazy loading is a design pattern that helps improve the performance of Angular applications by loading modules on demand, instead of loading everything upfront. This results in smaller initial bundles, faster load times, and improved user experiences.
In this guide, we will explore how lazy loading works in Angular, how to implement it, and its benefits.
1. How Lazy Loading Works in Angular
Lazy loading in Angular allows you to split your application into multiple feature modules, which are loaded only when needed. Instead of loading all modules at once when the app starts, Angular loads a module only when the user navigates to a route that uses it. This minimizes the initial load time and optimizes performance.
Lazy loading is particularly useful for large applications with many routes. By splitting the app into smaller modules, you ensure that only the necessary parts are loaded, improving the overall efficiency of your application.
Example: Basic Lazy Loading Setup
To set up lazy loading in Angular, you need to configure routes in your app-routing.module.ts file.
Old approach (without lazy loading):
import { NgModule } from '@angular/core';
import { RouterModule, Routes } from '@angular/router';
import { DashboardComponent } from './dashboard/dashboard.component';
import { SettingsComponent } from './settings/settings.component';
const routes: Routes = [
{ path: 'dashboard', component: DashboardComponent },
{ path: 'settings', component: SettingsComponent },
];
@NgModule({
imports: [RouterModule.forRoot(routes)],
exports: [RouterModule]
})
export class AppRoutingModule { }
New approach (with lazy loading):
import { NgModule } from '@angular/core';
import { RouterModule, Routes } from '@angular/router';
const routes: Routes = [
{
path: 'dashboard',
loadChildren: () => import('./dashboard/dashboard.module').then(m => m.DashboardModule)
},
{
path: 'settings',
loadChildren: () => import('./settings/settings.module').then(m => m.SettingsModule)
},
];
@NgModule({
imports: [RouterModule.forRoot(routes)],
exports: [RouterModule]
})
export class AppRoutingModule { }
2. Benefits of Lazy Loading
Lazy loading offers several advantages, especially for large-scale applications. Below are some key benefits:
- Improved Load Time: Only the necessary modules are loaded when required, reducing the initial bundle size and speeding up the app’s load time.
- Better Performance: Since Angular loads the code dynamically when the user navigates to a new route, the app remains performant even as it grows.
- Modular Code: Lazy loading encourages a modular architecture, making the app easier to maintain and scale over time.
- Reduced Memory Usage: As modules are loaded on demand, the app consumes less memory overall.
3. Configuring Preloading Strategies
While lazy loading improves performance, there are cases where you want to preload certain modules to enhance user experience. Angular offers various preloading strategies for better control.
Example: Preload All Modules
You can use Angular’s PreloadAllModules strategy to preload all lazy-loaded modules in the background, ensuring that they are available when the user navigates to their respective routes.
To set up a preloading strategy, modify your app-routing.module.ts as follows:
import { NgModule } from '@angular/core';
import { RouterModule, Routes } from '@angular/router';
import { PreloadAllModules } from '@angular/router';
const routes: Routes = [
{
path: 'dashboard',
loadChildren: () => import('./dashboard/dashboard.module').then(m => m.DashboardModule)
},
{
path: 'settings',
loadChildren: () => import('./settings/settings.module').then(m => m.SettingsModule)
},
];
@NgModule({
imports: [RouterModule.forRoot(routes, { preloadingStrategy: PreloadAllModules })],
exports: [RouterModule]
})
export class AppRoutingModule { }
4. Lazy Loading Guards
Sometimes, you may want to control whether a module is lazy-loaded based on certain conditions, such as user permissions. Angular provides guards like canLoad that allow you to restrict access to lazy-loaded modules.
Example: Using canLoad Guard
The canLoad guard checks if the module should be loaded before Angular triggers the lazy loading. This is useful for protecting sensitive routes that should only be accessed by authorized users.
Here’s an example of how to implement a canLoad guard:
import { Injectable } from '@angular/core';
import { CanLoad, Route, UrlSegment, Router } from '@angular/router';
import { AuthService } from './auth.service';
@Injectable({
providedIn: 'root',
})
export class AuthGuard implements CanLoad {
constructor(private authService: AuthService, private router: Router) {}
canLoad(route: Route, segments: UrlSegment[]): boolean {
if (this.authService.isLoggedIn()) {
return true;
} else {
this.router.navigate(['/login']);
return false;
}
}
}
// Applying the guard to a lazy-loaded route in app-routing.module.ts
const routes: Routes = [
{
path: 'admin',
loadChildren: () => import('./admin/admin.module').then(m => m.AdminModule),
canLoad: [AuthGuard]
},
];
Conclusion: Enhancing Angular Applications with Lazy Loading
Lazy loading is an essential optimization technique for large Angular applications. By loading modules on demand, you can reduce initial load times, improve performance, and enhance the user experience. Coupled with features like preloading strategies and guards, lazy loading offers fine-grained control over how and when parts of your application are loaded.
By leveraging lazy loading, developers can build scalable, high-performance Angular applications that deliver fast, efficient, and user-friendly experiences.