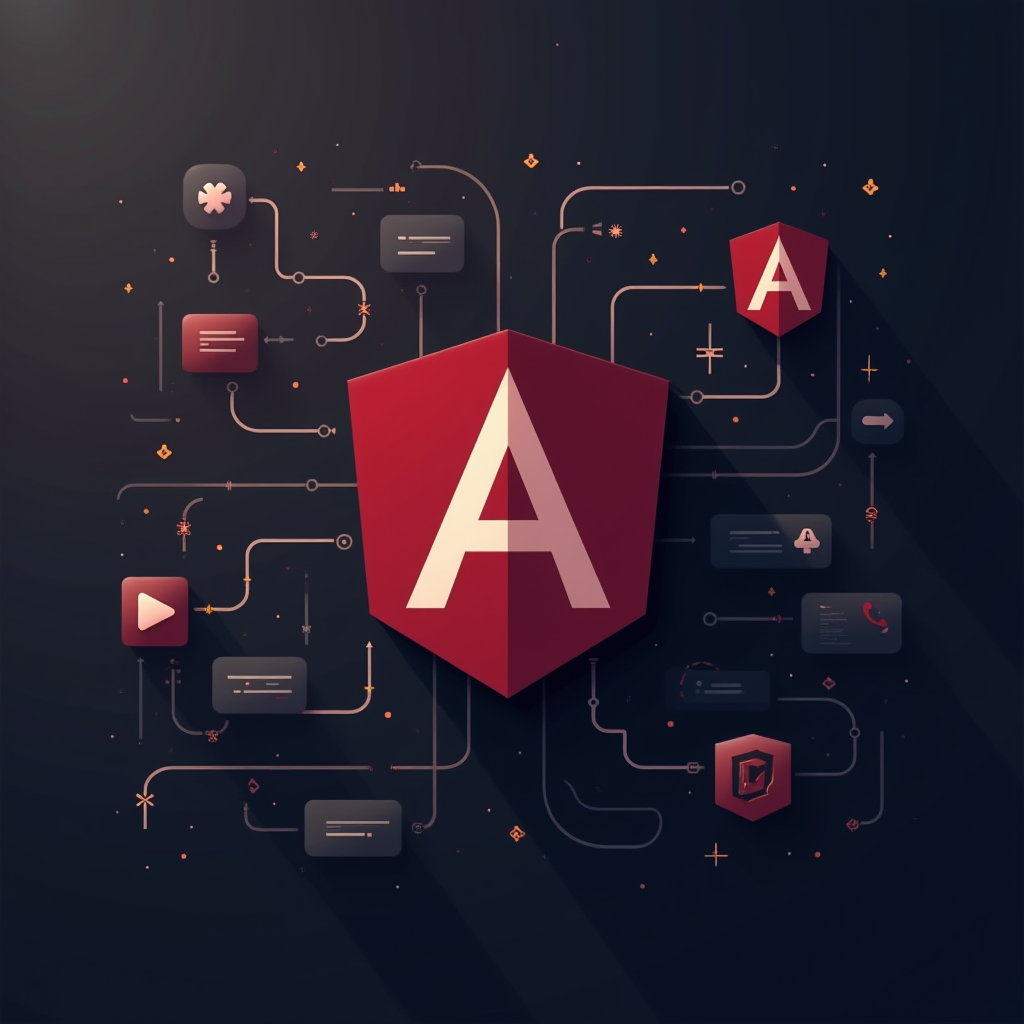
Mastering Control Flow in Angular: A Comprehensive Guide
Introduction: The Evolution of Control Flow in Angular
For years, Angular developers have relied on structural directives like *ngIf, *ngFor, and *ngSwitch to manage control flow in their templates. While powerful, these directives had limitations in readability and performance, especially in complex templates.
It is in this context that Angular introduced the new control flow blocks. This evolution addresses several crucial needs:
- More Intuitive Syntax: The new blocks @if, @for, and @switch provide syntax closer to native JavaScript, making the code easier to read and understand.
- Improved Performance: Unlike structural directives that require additional interpretation, control flow blocks are compiled directly, leading to better performance.
- No Need to Import CommonModule: A major advantage is that these new blocks do not require the import of CommonModule, simplifying your module structure.
- Better IDE Integration: The new blocks provide better integration with IDEs, facilitating autocompletion and error detection.
Let’s now explore these new control flow blocks in detail and compare them with their traditional counterparts.
1. Conditional Rendering with @if, @else-if, and @else
The @if block allows conditional rendering of content based on the evaluation of an expression.
Example: Basic Conditional Rendering
Old approach with *ngIf:
*ngIf="user.age >= 18">{{ user.name }} is an adult.
*ngIf="user.age < 18">{{ user.name }} is a minor.
New approach with @if:
@if (user.age >= 18) {
<p>{{ user.name }} is an adult.</p>
} @else {
<p>{{ user.name }} is a minor.</p>
}
Example: Multiple Conditions with @else-if
Old approach:
*ngIf="user.score > 90">{{ user.name }} has an excellent score!
*ngIf="user.score > 75 && user.score <= 90">{{ user.name }} has a good score.
*ngIf="user.score <= 75">{{ user.name }} needs improvement.
New approach:
@if (user.score > 90) {
<p>{{ user.name }} has an excellent score!</p>
} @else if (user.score > 75) {
<p>{{ user.name }} has a good score.</p>
} @else {
<p>{{ user.name }} needs improvement.</p>
}
2. Repeating Content with the @for Block
The @for block allows iterating over a collection and rendering content for each element.
Example: Basic Loop with @for
Old approach with *ngFor:
<ul>
*ngFor="let item of items; trackBy: trackById">{{ item.name }}</li>
</ul>
New approach with @for:
<ul>
@for (item of items; track item.id) {
<li>{{ item.name }}</li>
}
</ul>
The Importance of the track Expression
Using the track expression is crucial for performance, as it helps Angular efficiently manage DOM changes by correlating data with rendered elements.
Example: Optimized Loop with Tracking and Fallback Content
Old approach:
<ul>
*ngFor="let item of items; trackBy: trackById">{{ item.name }}</li>
*ngIf="items.length === 0">No items available.</li>
</ul>
New approach:
<ul>
@for (item of items; track item.id) {
<li>{{ item.name }}</li>
} @empty {
<li>No items available.</li>
}
</ul>
3. Conditional Rendering with the @switch Block
The @switch block offers an alternative syntax for conditional rendering.
Example: Using @switch for Role-Based Content
Old approach with *ngSwitch:
<ng-container [ngSwitch]="user.role">
<app-admin-dashboard *ngSwitchCase="'admin'"></app-admin-dashboard>
<app-editor-dashboard *ngSwitchCase="'editor'"></app-editor-dashboard>
<app-viewer-dashboard *ngSwitchCase="'viewer'"></app-viewer-dashboard>
<p *ngSwitchDefault>You do not have permission to access this content.</p>
</ng-container>
New approach with @switch:
@switch (user.role) {
@case ('admin') {
<app-admin-dashboard />
}
@case ('editor') {
<app-editor-dashboard />
}
@case ('viewer') {
<app-viewer-dashboard />
}
@default {
<p>You do not have permission to access this content.</p>
}
}
Summary and Performance Considerations
Angular's new control flow blocks offer several significant advantages:
- Clearer and More Intuitive Syntax: The new syntax is closer to native JavaScript, making the code easier to read and maintain.
- Better Performance: Control flow blocks are compiled directly, leading to better performance compared to traditional structural directives.
- Reduced Bundle Size: The lack of need to import CommonModule can lead to a reduction in your application's final bundle size.
- Better Development Tool Support: The new blocks provide better support from IDEs, making error detection and autocompletion easier.
By adopting these new control flow blocks, Angular developers can create more expressive, performant, and maintainable templates. While the transition may require some adjustment time, the benefits in code clarity and performance make it a welcome change in the Angular ecosystem.